class: center, middle, inverse, title-slide # Parallel Iterations ### Daniel Anderson ### Week 5, Class 1 --- layout: true <script> feather.replace() </script> <div class="slides-footer"> <span> <a class = "footer-icon-link" href = "https://github.com/uo-datasci-specialization/c3-fp-2021/raw/main/static/slides/w5p1.pdf"> <i class = "footer-icon" data-feather="download"></i> </a> <a class = "footer-icon-link" href = "https://fp-2021.netlify.app/slides/w5p1.html"> <i class = "footer-icon" data-feather="link"></i> </a> <a class = "footer-icon-link" href = "https://fp-2021.netlify.app/"> <i class = "footer-icon" data-feather="globe"></i> </a> <a class = "footer-icon-link" href = "https://github.com/uo-datasci-specialization/c3-fp-2021"> <i class = "footer-icon" data-feather="github"></i> </a> </span> </div> --- # Agenda * Finish up slides from last week * Discuss `map2_*` and `pmap_*` --- # Learning objectives * Understand the differences between `map`, `map2`, and `pmap` --- background-image:url(https://d33wubrfki0l68.cloudfront.net/7a545699ff7069a98329fcfbe6e42b734507eb16/211a5/diagrams/functionals/map2-arg.png) background-size:contain # `map2` --- class: inverse-blue middle # A few Examples Basic simulations - iterating over two vectors Plots by month, changing the title --- # Simulation * Simulate data from a normal distribution + Vary `\(n\)` from 5 to 150 by increments of 5 -- + For each `\(n\)`, vary `\(mu\)` from -2 to 2 by increments of 0.25 -- ### How do we get all combinations -- `expand.grid` --- # Example `expand.grid` ### Bonus: It turns it into a data frame! ```r ints <- 1:3 lets <- c("a", "b", "c") expand.grid(ints, lets) ``` ``` ## Var1 Var2 ## 1 1 a ## 2 2 a ## 3 3 a ## 4 1 b ## 5 2 b ## 6 3 b ## 7 1 c ## 8 2 c ## 9 3 c ``` --- # Set conditions Please follow along ```r conditions <- expand.grid(n = seq(5, 150, 5), mu = seq(-2, 2, 0.25)) head(conditions) ``` ``` ## n mu ## 1 5 -2 ## 2 10 -2 ## 3 15 -2 ## 4 20 -2 ## 5 25 -2 ## 6 30 -2 ``` ```r tail(conditions) ``` ``` ## n mu ## 505 125 2 ## 506 130 2 ## 507 135 2 ## 508 140 2 ## 509 145 2 ## 510 150 2 ``` --- # Simulate! ```r sim1 <- map2(conditions$n, conditions$mu, ~{ rnorm(n = .x, mean = .y, sd = 10) }) str(sim1) ``` ``` ## List of 510 ## $ : num [1:5] -8.89 11.59 -6.16 -7.1 3.81 ## $ : num [1:10] -5.967 -14.048 -0.242 -17.481 8.045 ... ## $ : num [1:15] 5.103 -0.816 -5.772 -13.166 10.696 ... ## $ : num [1:20] 3.3 -14.74 -4.39 7.39 -20.82 ... ## $ : num [1:25] -5.64 17.8 -12.89 1.92 -1.03 ... ## $ : num [1:30] -4.47 7.7 3.9 -3.88 10.41 ... ## $ : num [1:35] 11.3211 5.2912 -0.0202 0.7237 2.5658 ... ## $ : num [1:40] -5.36 14.02 1.73 -3.9 -6.73 ... ## $ : num [1:45] 1.32 13.89 19.73 4.62 -16.47 ... ## $ : num [1:50] -19.54 -1.02 -6.72 -8.03 12.07 ... ## $ : num [1:55] -3.96 -2.31 -6.94 17.01 6.67 ... ## $ : num [1:60] 0.169 6.871 -0.963 1.925 -6.045 ... ## $ : num [1:65] 10.64 -16.511 -0.575 -6.33 -15.544 ... ## $ : num [1:70] 4.84 -1.43 9.39 9.09 22.67 ... ## $ : num [1:75] -7.264 0.494 -10.131 -15.417 -12.42 ... ## $ : num [1:80] -4.49 5.16 -16.3 3.68 -5.99 ... ## $ : num [1:85] -7 20.57 -3.59 -10.93 -6.08 ... ## $ : num [1:90] 1.27 -4.96 7.39 10.66 -1.2 ... ## $ : num [1:95] -0.562 -16.97 10.457 -0.644 -1.726 ... ## $ : num [1:100] -7.589 0.757 -7.241 -19.506 -1.601 ... ## $ : num [1:105] 8.71 -1.01 -18.79 -2.35 -7.49 ... ## $ : num [1:110] 3.1764 -6.171 12.3225 -1.2728 -0.0135 ... ## $ : num [1:115] 11.52 -13.41 -20.38 10.52 -5.49 ... ## $ : num [1:120] 0.756 -3.554 3.401 10.524 4.408 ... ## $ : num [1:125] -0.694 18.045 -8.461 -11.264 -0.76 ... ## $ : num [1:130] -11.984 -0.623 6.513 8.497 -5.168 ... ## $ : num [1:135] -21.403 -11.645 -9.269 -5.855 0.682 ... ## $ : num [1:140] 1.07 24.46 -8.59 4.96 -7.9 ... ## $ : num [1:145] -7.9 22.38 4.88 -17.46 -2.74 ... ## $ : num [1:150] 10.382 1.299 5.264 9.303 0.339 ... ## $ : num [1:5] -15.64 4 4.01 2.83 -10.27 ## $ : num [1:10] -8.106 2.349 0.694 -2.7 15.969 ... ## $ : num [1:15] -11.2 4.66 -8.72 1.45 -11.5 ... ## $ : num [1:20] 5.59 6.56 2.8 -11.09 -5.93 ... ## $ : num [1:25] -1.21 -8.23 7.41 -12.76 -19.67 ... ## $ : num [1:30] 8.88 7.94 -11.45 -14.62 -10.42 ... ## $ : num [1:35] 1.784 -13.075 -0.478 -0.291 -11.102 ... ## $ : num [1:40] -6.31 -10.05 16.66 14.96 -26.49 ... ## $ : num [1:45] 8.83 -14.74 -8.99 -2.57 -1.22 ... ## $ : num [1:50] -1.15 0.95 21.68 11.86 8.68 ... ## $ : num [1:55] 5.36 5.78 -15.29 -25.96 35.88 ... ## $ : num [1:60] -16.84 -5.44 23.98 -15.03 11.3 ... ## $ : num [1:65] -0.488 8.281 -6.468 -2.943 -8.026 ... ## $ : num [1:70] 2.93 19.19 -9.55 1.23 -14.18 ... ## $ : num [1:75] -13.933 -8.524 -13.295 0.174 -1.812 ... ## $ : num [1:80] -7.776 8.925 11.965 -9.403 0.907 ... ## $ : num [1:85] 1 7.14 8.37 7.58 4.64 ... ## $ : num [1:90] -8.472 -22.605 -0.167 7.205 3.682 ... ## $ : num [1:95] -18.543 3.599 -4.158 0.354 16.679 ... ## $ : num [1:100] -9.67 -1.67 -17.5 2.63 -8.83 ... ## $ : num [1:105] -17.43 12.64 -26.39 -7.97 -7.1 ... ## $ : num [1:110] -7.33 -5.85 2.23 11.65 2.53 ... ## $ : num [1:115] -5.82 -2.02 -17.5 -15.41 1.88 ... ## $ : num [1:120] 11.1 -16.58 -3.48 13.61 -14.24 ... ## $ : num [1:125] 3.865 15.589 5.162 12.538 0.507 ... ## $ : num [1:130] 16.49 17.13 -12.78 -2.82 9.04 ... ## $ : num [1:135] 4.446 7.547 -0.351 15.577 14.497 ... ## $ : num [1:140] -1.18 5.34 3.57 -1.66 -4.78 ... ## $ : num [1:145] -11.33 10 -4.82 -17.41 -2.2 ... ## $ : num [1:150] -10.78 -8.64 -13.54 10.11 2.79 ... ## $ : num [1:5] -4.18 2.32 5.3 -21.26 -3.18 ## $ : num [1:10] -1.2 4.28 -11.33 6.81 2.87 ... ## $ : num [1:15] 4.76 6.38 1.89 -1.25 -5.29 ... ## $ : num [1:20] -1.42 11.05 -7.19 -7.92 3.51 ... ## $ : num [1:25] 4.19 -6.71 11.5 -16.6 -6.23 ... ## $ : num [1:30] -14.55 -2.81 27.39 -11.79 -0.21 ... ## $ : num [1:35] -0.253 -0.412 2.388 -13.155 -13.82 ... ## $ : num [1:40] -0.645 -6.149 5.5 4.469 2.458 ... ## $ : num [1:45] -5.22 12.14 -16.84 -29.1 16.07 ... ## $ : num [1:50] 4.395 -1.531 0.943 11.338 0.676 ... ## $ : num [1:55] 11.37 0.406 16.455 1.067 10.846 ... ## $ : num [1:60] -14.41 15.92 8.45 -3.95 -2.15 ... ## $ : num [1:65] -20.1 -3.08 4.21 -14.95 -1.5 ... ## $ : num [1:70] 17.62 -1.94 -27.02 12.75 -7.39 ... ## $ : num [1:75] -6.24 -4.78 6.29 -17.02 1.21 ... ## $ : num [1:80] 5.35 2.4 -11.44 -16.15 3.97 ... ## $ : num [1:85] 13.676 11.162 20.833 0.483 7.093 ... ## $ : num [1:90] 2.9 2.78 -19.45 -1.66 -4.31 ... ## $ : num [1:95] -7.05 2.94 -16.43 -6.69 -7.67 ... ## $ : num [1:100] 2.86 -11 -14.43 -12.48 -1.75 ... ## $ : num [1:105] 8.283 -8.015 -0.538 1.435 -0.652 ... ## $ : num [1:110] -19.57 -5.17 -0.63 -10.41 -9.36 ... ## $ : num [1:115] -9.057 -4.536 -16.9 0.344 3.115 ... ## $ : num [1:120] -6.55 -6.24 -8.16 -5.36 3.27 ... ## $ : num [1:125] 7.04 -9.77 10.9 -12.86 -3.91 ... ## $ : num [1:130] -5.82 -15.64 3.57 5.93 -1.12 ... ## $ : num [1:135] 11.79 2.96 10.5 -10.76 20.74 ... ## $ : num [1:140] 17.3 -13.55 -17.74 1.35 -1.3 ... ## $ : num [1:145] -4.29 7.35 -1.4 6.87 -12.03 ... ## $ : num [1:150] 22.11 3.6 -6.21 -3.42 -9.64 ... ## $ : num [1:5] -7.7 -6.975 -0.467 -30.599 2.568 ## $ : num [1:10] 12.76 3.38 1.68 9.99 -7.66 ... ## $ : num [1:15] -8.78 -6.38 10.45 -11.93 11.27 ... ## $ : num [1:20] 12.53 9.32 -1.09 -1.62 -6.46 ... ## $ : num [1:25] -4.93 3.37 -16.83 -5.97 11.93 ... ## $ : num [1:30] 1.5 3.81 -12.67 11.05 17.08 ... ## $ : num [1:35] -13.17 6 6.27 -15.47 -4.61 ... ## $ : num [1:40] -23.094 -3.444 0.187 3.589 0.726 ... ## $ : num [1:45] -5.35 -1.12 -4.32 -2.25 12.18 ... ## [list output truncated] ``` --- # More powerful ### Add it as a list column! ```r sim2 <- conditions %>% as_tibble() %>% # Not required, but definitely helpful mutate(sim = map2(n, mu, ~rnorm(n = .x, mean = .y, sd = 10))) sim2 ``` ``` ## # A tibble: 510 x 3 ## n mu sim ## <dbl> <dbl> <list> ## 1 5 -2 <dbl [5]> ## 2 10 -2 <dbl [10]> ## 3 15 -2 <dbl [15]> ## 4 20 -2 <dbl [20]> ## 5 25 -2 <dbl [25]> ## 6 30 -2 <dbl [30]> ## 7 35 -2 <dbl [35]> ## 8 40 -2 <dbl [40]> ## 9 45 -2 <dbl [45]> ## 10 50 -2 <dbl [50]> ## # … with 500 more rows ``` --- # Unnest ```r conditions %>% as_tibble() %>% mutate(sim = map2(n, mu, ~rnorm(.x, .y, sd = 10))) %>% unnest(sim) ``` ``` ## # A tibble: 39,525 x 3 ## n mu sim ## <dbl> <dbl> <dbl> ## 1 5 -2 7.570682 ## 2 5 -2 -2.268110 ## 3 5 -2 -11.52502 ## 4 5 -2 -5.372831 ## 5 5 -2 -4.913946 ## 6 10 -2 0.5334139 ## 7 10 -2 6.504093 ## 8 10 -2 -20.98906 ## 9 10 -2 13.81959 ## 10 10 -2 -16.19086 ## # … with 39,515 more rows ``` --- # Challenge Can you replicate what we just did, but using a `rowwise()` approach?
03
:
00
-- ```r conditions %>% rowwise() %>% mutate(sim = list(rnorm(n, mu, sd = 10))) %>% unnest(sim) ``` ``` ## # A tibble: 39,525 x 3 ## n mu sim ## <dbl> <dbl> <dbl> ## 1 5 -2 13.95355 ## 2 5 -2 31.02931 ## 3 5 -2 -2.671605 ## 4 5 -2 -9.867195 ## 5 5 -2 -2.897235 ## 6 10 -2 -19.70467 ## 7 10 -2 10.80861 ## 8 10 -2 7.158649 ## 9 10 -2 2.255881 ## 10 10 -2 5.924527 ## # … with 39,515 more rows ``` --- class: inverse-orange middle # Vary the sd too? -- ### `pmap` Which we'll get to soon --- class: inverse-blue middle # Varying the title of a plot --- # The data Please follow along ```r library(fivethirtyeight) pulitzer ``` ``` ## # A tibble: 50 x 7 ## newspaper circ2004 circ2013 pctchg_circ num_finals1990_2003 num_finals2004_2014 ## <chr> <dbl> <dbl> <int> <int> <int> ## 1 USA Today 2192098 1674306 -24 1 1 ## 2 Wall Street Journal 2101017 2378827 13 30 20 ## 3 New York Times 1119027 1865318 67 55 62 ## 4 Los Angeles Times 983727 653868 -34 44 41 ## 5 Washington Post 760034 474767 -38 52 48 ## 6 New York Daily News 712671 516165 -28 4 2 ## 7 New York Post 642844 500521 -22 0 0 ## 8 Chicago Tribune 603315 414930 -31 23 15 ## 9 San Jose Mercury News 558874 583998 4 4 2 ## 10 Newsday 553117 377744 -32 12 6 ## # … with 40 more rows, and 1 more variable: num_finals1990_2014 <int> ``` --- # Prep data ```r pulitzer<- pulitzer %>% select(newspaper, starts_with("num")) %>% pivot_longer( -newspaper, names_to = "year_range", values_to = "n", names_prefix = "num_finals" ) %>% mutate(year_range = str_replace_all(year_range, "_", "-")) %>% filter(year_range != "1990-2014") head(pulitzer) ``` ``` ## # A tibble: 6 x 3 ## newspaper year_range n ## <chr> <chr> <int> ## 1 USA Today 1990-2003 1 ## 2 USA Today 2004-2014 1 ## 3 Wall Street Journal 1990-2003 30 ## 4 Wall Street Journal 2004-2014 20 ## 5 New York Times 1990-2003 55 ## 6 New York Times 2004-2014 62 ``` --- # One plot ```r pulitzer%>% filter(newspaper == "Wall Street Journal") %>% ggplot(aes(n, year_range)) + geom_col(aes(fill = n)) + scale_fill_distiller(type = "seq", limits = c(0, max(pulitzer$n)), palette = "BuPu", direction = 1) + scale_x_continuous(limits = c(0, max(pulitzer$n)), expand = c(0, 0)) + guides(fill = "none") + labs(title = "Pulitzer Prize winners: Wall Street Journal", x = "Total number of winners", y = "") ``` 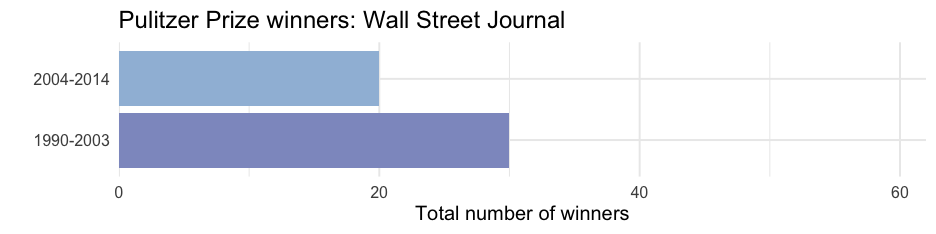<!-- --> --- # Nest data ```r pulitzer%>% group_by(newspaper) %>% nest() ``` ``` ## # A tibble: 50 x 2 ## # Groups: newspaper [50] ## newspaper data ## <chr> <list> ## 1 USA Today <tibble[,2] [2 × 2]> ## 2 Wall Street Journal <tibble[,2] [2 × 2]> ## 3 New York Times <tibble[,2] [2 × 2]> ## 4 Los Angeles Times <tibble[,2] [2 × 2]> ## 5 Washington Post <tibble[,2] [2 × 2]> ## 6 New York Daily News <tibble[,2] [2 × 2]> ## 7 New York Post <tibble[,2] [2 × 2]> ## 8 Chicago Tribune <tibble[,2] [2 × 2]> ## 9 San Jose Mercury News <tibble[,2] [2 × 2]> ## 10 Newsday <tibble[,2] [2 × 2]> ## # … with 40 more rows ``` --- # Produce all plots ### You try first! Don't worry about the correct title yet, if you don't want
03
:
00
-- ```r pulitzer%>% group_by(newspaper) %>% nest() %>% mutate(plot = map(data, ~{ ggplot(aes(n, year_range)) + geom_col(aes(fill = n)) + scale_fill_distiller(type = "seq", limits = c(0, max(pulitzer$n)), palette = "BuPu", direction = 1) + scale_x_continuous(limits = c(0, max(pulitzer$n)), expand = c(0, 0)) + guides(fill = "none") + labs(title = "Pulitzer Prize winners", x = "Total number of winners", y = "") }) ) ``` --- # Add title ```r library(glue) p <- pulitzer%>% group_by(newspaper) %>% nest() %>% *mutate(plot = map2(data, newspaper, ~{ ggplot(.x, aes(n, year_range)) + geom_col(aes(fill = n)) + scale_fill_distiller(type = "seq", limits = c(0, max(pulitzer$n)), palette = "BuPu", direction = 1) + scale_x_continuous(limits = c(0, max(pulitzer$n)), expand = c(0, 0)) + guides(fill = "none") + * labs(title = glue("Pulitzer Prize winners: {.y}"), x = "Total number of winners", y = "") }) ) ``` --- ```r p ``` ``` ## # A tibble: 50 x 3 ## # Groups: newspaper [50] ## newspaper data plot ## <chr> <list> <list> ## 1 USA Today <tibble[,2] [2 × 2]> <gg> ## 2 Wall Street Journal <tibble[,2] [2 × 2]> <gg> ## 3 New York Times <tibble[,2] [2 × 2]> <gg> ## 4 Los Angeles Times <tibble[,2] [2 × 2]> <gg> ## 5 Washington Post <tibble[,2] [2 × 2]> <gg> ## 6 New York Daily News <tibble[,2] [2 × 2]> <gg> ## 7 New York Post <tibble[,2] [2 × 2]> <gg> ## 8 Chicago Tribune <tibble[,2] [2 × 2]> <gg> ## 9 San Jose Mercury News <tibble[,2] [2 × 2]> <gg> ## 10 Newsday <tibble[,2] [2 × 2]> <gg> ## # … with 40 more rows ``` --- # Look at a couple plots .pull-left[ ```r p$plot[[1]] ``` 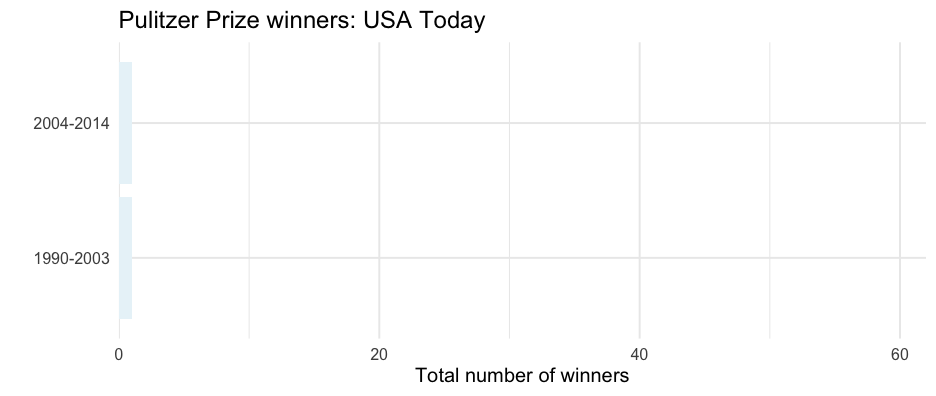<!-- --> ```r p$plot[[3]] ``` 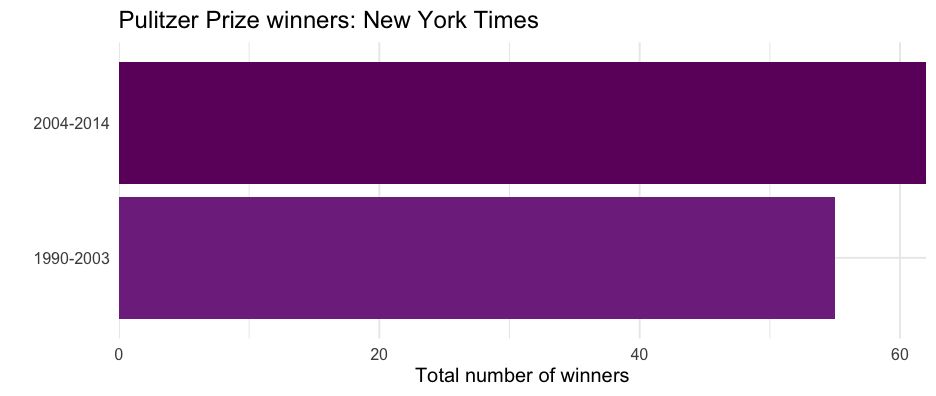<!-- --> ] .pull-right[ ```r p$plot[[2]] ``` 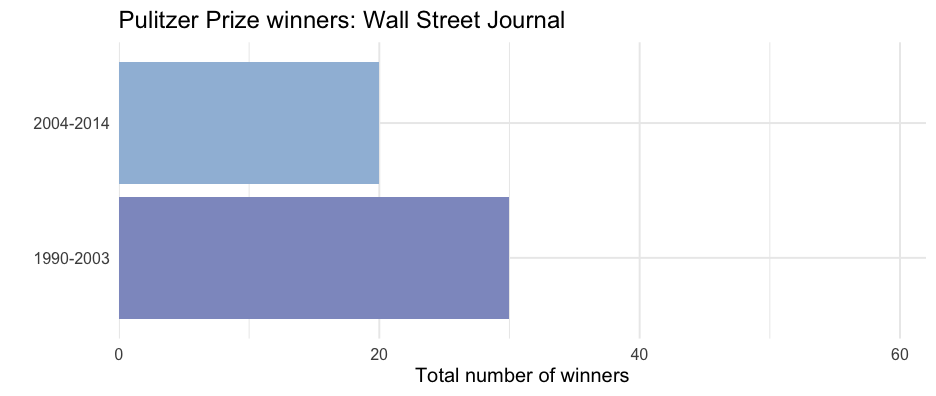<!-- --> ```r p$plot[[4]] ``` 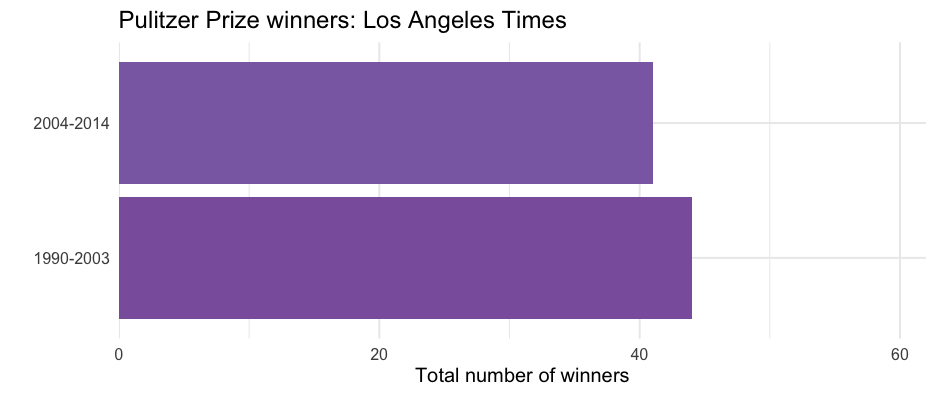<!-- --> ] --- # Challenge (You can probably guess where this is going) -- ## Can you reproduce the prior plots using a `rowwise()` approach? --- ```r pulitzer%>% *nest_by(newspaper) %>% mutate( plot = list( * ggplot(data, aes(n, year_range)) + geom_col(aes(fill = n)) + scale_fill_distiller(type = "seq", limits = c(0, max(pulitzer$n)), palette = "BuPu", direction = 1) + scale_x_continuous(limits = c(0, max(pulitzer$n)), expand = c(0, 0)) + guides(fill = "none") + * labs(title = glue("Pulitzer Prize winners: {newspaper}"), x = "Total number of winners", y = "") ) ) ``` ``` ## # A tibble: 50 x 3 ## # Rowwise: newspaper ## newspaper data plot ## <chr> <list<tibble[,2]>> <list> ## 1 Arizona Republic [2 × 2] <gg> ## 2 Atlanta Journal Constitution [2 × 2] <gg> ## 3 Baltimore Sun [2 × 2] <gg> ## 4 Boston Globe [2 × 2] <gg> ## 5 Boston Herald [2 × 2] <gg> ## 6 Charlotte Observer [2 × 2] <gg> ## 7 Chicago Sun-Times [2 × 2] <gg> ## 8 Chicago Tribune [2 × 2] <gg> ## 9 Cleveland Plain Dealer [2 × 2] <gg> ## 10 Columbus Dispatch [2 × 2] <gg> ## # … with 40 more rows ``` --- class: inverse-red middle # Iterating over `\(n\)` vectors ### `pmap` --- background-image:url(https://d33wubrfki0l68.cloudfront.net/e426c5755e2e65bdcc073d387775db79791f32fd/92902/diagrams/functionals/pmap.png) background-size:contain # `pmap` --- # Simulation * Simulate data from a normal distribution + Vary `\(n\)` from 5 to 150 by increments of 5 -- + For each `\(n\)`, vary `\(mu\)` from -2 to 2 by increments of 0.25 -- + For each `\(\sigma\)` from 1 to 3 by increments of 0.1 --- ```r full_conditions <- expand.grid(n = seq(5, 150, 5), mu = seq(-2, 2, 0.25), sd = seq(1, 3, .1)) head(full_conditions) ``` ``` ## n mu sd ## 1 5 -2 1 ## 2 10 -2 1 ## 3 15 -2 1 ## 4 20 -2 1 ## 5 25 -2 1 ## 6 30 -2 1 ``` ```r tail(full_conditions) ``` ``` ## n mu sd ## 10705 125 2 3 ## 10706 130 2 3 ## 10707 135 2 3 ## 10708 140 2 3 ## 10709 145 2 3 ## 10710 150 2 3 ``` --- # Full Simulation ```r fsim <- pmap( list(number = full_conditions$n, average = full_conditions$mu, stdev = full_conditions$sd), function(number, average, stdev) { rnorm(n = number, mean = average, sd = stdev) } ) str(fsim) ``` ``` ## List of 10710 ## $ : num [1:5] -1.536 -2.266 -1.205 -2.234 -0.944 ## $ : num [1:10] -0.841 -2.637 -2.427 -1.969 -2.696 ... ## $ : num [1:15] -2.397 -1.581 -1.349 -1.856 0.587 ... ## $ : num [1:20] -1.767 -3.026 -1.364 -2.747 -0.197 ... ## $ : num [1:25] -2.56 -2.13 -1.33 -2.9 -3.86 ... ## $ : num [1:30] -3.95 -1.88 -1.21 -1.5 -1.5 ... ## $ : num [1:35] -1.56 -1.69 -4.14 -3.05 -1.93 ... ## $ : num [1:40] -2.979 -1.432 -1.607 -1.905 -0.628 ... ## $ : num [1:45] -1.829 -1.772 -1.432 -0.315 -2.345 ... ## $ : num [1:50] -2.25 -1.93 -3.97 -1.59 -2.74 ... ## $ : num [1:55] -3.562 -0.966 -1.718 -1.661 -1.809 ... ## $ : num [1:60] -1.35 -1.15 -1.74 -2.3 -3.64 ... ## $ : num [1:65] -2.47 -1.24 -2.43 -2.27 -2.34 ... ## $ : num [1:70] -1.349 -2.941 -0.651 -2.054 -1.731 ... ## $ : num [1:75] -2.262 -1.153 -2.463 -0.941 -2.026 ... ## $ : num [1:80] -0.862 -1.633 -0.92 -1.897 -0.685 ... ## $ : num [1:85] -2.82 -1.138 -0.755 -1.535 -2.639 ... ## $ : num [1:90] -2.89 -0.924 -2.09 -1.869 -1.388 ... ## $ : num [1:95] -3.61 -2.58 -2.46 -2.15 -2.22 ... ## $ : num [1:100] -3.74 -1.3 -2.5 -2.19 -1.95 ... ## $ : num [1:105] -2.52 -2.337 -0.567 -1.741 -3.519 ... ## $ : num [1:110] -3.96 -2.1 -1.03 -2.82 -2.35 ... ## $ : num [1:115] -0.819 -2.511 -2.104 0.287 -1.549 ... ## $ : num [1:120] -1.39 -2.06 -1.83 -2.53 -2.2 ... ## $ : num [1:125] -0.98651 -0.05067 -2.90015 0.00538 -2.95578 ... ## $ : num [1:130] -1.129 -1.764 -0.516 -1.5 -3.097 ... ## $ : num [1:135] -2.417 -2.952 -1.637 -2.34 -0.543 ... ## $ : num [1:140] -2.4 -2.578 -1.755 -2.448 -0.388 ... ## $ : num [1:145] -1.37 -2.65 -1.09 -1.27 -2.24 ... ## $ : num [1:150] -0.807 -4.206 -3.005 -2.991 -1.578 ... ## $ : num [1:5] -2.263 -1.338 -0.222 -1.286 -2.412 ## $ : num [1:10] -2.155 0.857 0.579 -0.713 -1.845 ... ## $ : num [1:15] -0.896 -0.965 0.598 -0.374 -2.154 ... ## $ : num [1:20] -2.078 -1.458 -1.439 -0.411 -2.641 ... ## $ : num [1:25] -1.441 -1.34 0.311 -2.675 -1.602 ... ## $ : num [1:30] -0.955 -1.28 -2.141 0.261 -2.227 ... ## $ : num [1:35] -1.829 1.785 -0.205 -1.888 -2.248 ... ## $ : num [1:40] -4.433 -0.982 -4.026 -1.432 -1.963 ... ## $ : num [1:45] -2.429 -2.694 -1.752 -1.913 -0.509 ... ## $ : num [1:50] -3.4296 -1.5497 -1.394 0.0127 -2.3191 ... ## $ : num [1:55] -1.368 -0.958 -1.292 -2.09 0.502 ... ## $ : num [1:60] -3.716 -2.458 -1.584 -1.055 -0.939 ... ## $ : num [1:65] -1.709 -0.466 -2.235 -2.929 -0.658 ... ## $ : num [1:70] -2.693 -0.761 -1.057 -0.342 0.492 ... ## $ : num [1:75] -1.79 -2.4 -1.08 -1.79 -3.38 ... ## $ : num [1:80] -2.2032 -1.8056 -2.5973 -1.5846 -0.0132 ... ## $ : num [1:85] -2.4 -1.24 -1.78 -2.58 -2 ... ## $ : num [1:90] -0.497 -1.768 -2.999 -1.153 -3.143 ... ## $ : num [1:95] -1.902 -3.569 -0.919 0.559 -1.739 ... ## $ : num [1:100] -2.62 -1.88 -3 -1.68 -1.14 ... ## $ : num [1:105] -2.06 -1.75 -1.34 -2.44 -1.79 ... ## $ : num [1:110] 0.189 -3.496 -2.917 -3.467 -2.244 ... ## $ : num [1:115] -1.071 -1.245 -3.165 -0.424 -2.995 ... ## $ : num [1:120] -0.82 -1.886 -2.771 -2.312 -0.653 ... ## $ : num [1:125] -1.09 -1.82 -1.25 -2.06 -1.21 ... ## $ : num [1:130] -2.161 -3.677 -0.812 -1.201 -0.453 ... ## $ : num [1:135] -4.855 -0.877 -1.915 -3.513 -1.959 ... ## $ : num [1:140] -1.353 -0.682 -1.759 -2.018 -0.129 ... ## $ : num [1:145] -2.56 -1.59 -2.67 -3.09 -1.71 ... ## $ : num [1:150] -1.65 -1.56 -1.9 -2.06 -1.36 ... ## $ : num [1:5] -1.26 -1.26 -2.27 -1.97 -0.6 ## $ : num [1:10] -2.225 -1.227 -1.044 -0.568 -1.137 ... ## $ : num [1:15] -1.81 -2.06 -2.22 -1.35 -2.94 ... ## $ : num [1:20] -2.26 -1.72 -1.47 -1.74 -2.59 ... ## $ : num [1:25] -1.36 -1.29 -3.56 -2.96 -3.11 ... ## $ : num [1:30] 0.0545 -0.9368 -2.6742 -0.2815 -0.112 ... ## $ : num [1:35] -1.404 -0.526 -3.585 -1.257 -2.43 ... ## $ : num [1:40] -2.51 -1.53 -1.49 -1.68 -1.45 ... ## $ : num [1:45] -0.806 -1.678 -1.745 -2.101 -0.455 ... ## $ : num [1:50] -0.388 0.121 -2.335 -2.316 0.194 ... ## $ : num [1:55] -1.65 -2.55 -1.92 -2.17 -4.24 ... ## $ : num [1:60] -1.917 -0.577 -1.115 -2.226 -2.317 ... ## $ : num [1:65] 0.482 -0.792 0.225 -2.623 -2.502 ... ## $ : num [1:70] -0.768 -0.491 -0.996 -1.195 -1.094 ... ## $ : num [1:75] -1.434 -2.759 -2.471 -1.138 0.346 ... ## $ : num [1:80] 0.301 -1.918 -0.6 -2.349 -0.694 ... ## $ : num [1:85] -2.56 -1.51 -1.73 -1.43 -1.74 ... ## $ : num [1:90] -0.843 -1.781 -2.555 -1.108 -1.852 ... ## $ : num [1:95] -1.898 -1.193 -2.47 -2.968 -0.565 ... ## $ : num [1:100] -2.6637 -0.0529 -2.4872 -1.6588 -1.3545 ... ## $ : num [1:105] -2.82 -2.25 -3 -1.91 -2.15 ... ## $ : num [1:110] -1.478 -1.774 -0.072 -2.056 -2.089 ... ## $ : num [1:115] -1.79 -0.582 -0.232 -2.57 -1.586 ... ## $ : num [1:120] -2.04 -1.806 -0.234 -0.287 -1.114 ... ## $ : num [1:125] -0.2575 -0.5272 -2.1321 -1.2277 0.0714 ... ## $ : num [1:130] -2.1145 -0.9458 -1.3888 -2.4251 0.0279 ... ## $ : num [1:135] -0.523 -1.32 -1.951 -1.915 0.564 ... ## $ : num [1:140] -0.208 -1.821 -1.85 -2.347 -0.99 ... ## $ : num [1:145] -1.654 -1.422 -1.579 -1.979 0.136 ... ## $ : num [1:150] -0.534 -1.139 -2.15 -0.905 -1.074 ... ## $ : num [1:5] -1.783 -0.155 -0.386 -1.179 0.557 ## $ : num [1:10] 1.26 -1.07 -2.07 -1.57 -2.47 ... ## $ : num [1:15] -0.786 -0.522 0.468 -1.124 -0.967 ... ## $ : num [1:20] 0.0891 -1.575 -1.7176 -1.871 -2.6302 ... ## $ : num [1:25] -3.389 -0.322 -1.518 -0.846 -0.735 ... ## $ : num [1:30] 0.79 -1.901 -2.854 -0.12 -0.397 ... ## $ : num [1:35] 0.259 -0.37 -1.102 -1.955 -1.142 ... ## $ : num [1:40] -0.806 -2.416 -1.666 -1.395 -2.467 ... ## $ : num [1:45] -1.868 -0.112 -0.217 -1.265 -2.04 ... ## [list output truncated] ``` --- # Alternative spec ```r fsim <- pmap(list(full_conditions$n, full_conditions$mu, full_conditions$sd), ~rnorm(n = ..1, mean = ..2, sd = ..3)) str(fsim) ``` ``` ## List of 10710 ## $ : num [1:5] -2.15 -2.45 -2.93 -3.36 -1.7 ## $ : num [1:10] -1.777 -0.882 -2.075 -2.826 -2.757 ... ## $ : num [1:15] -1.52044 -1.45154 -0.30679 -0.16667 -0.00603 ... ## $ : num [1:20] -0.627 -1.191 -0.71 -2.197 -1.363 ... ## $ : num [1:25] -1.7 -1.83 -1.86 -2.42 -2.94 ... ## $ : num [1:30] -1.32 -2.53 -1.6 -1.02 -3.23 ... ## $ : num [1:35] 0.0321 -0.798 -1.0541 -1.5625 -0.2858 ... ## $ : num [1:40] -2.44 -1.53 -2.03 -2.34 -2.6 ... ## $ : num [1:45] -2.22 -2.16 -3.81 -2.88 -0.73 ... ## $ : num [1:50] -1.81 -3.08 -1.15 -2.61 -2.3 ... ## $ : num [1:55] -1.87 -2.224 -3.966 -0.403 -2.465 ... ## $ : num [1:60] -2.86 -2.27 -2.95 -2.23 -3.99 ... ## $ : num [1:65] 0.54 -1.2 -0.74 -2.49 -2.55 ... ## $ : num [1:70] -0.342 -3.39 -2.711 0.766 -0.39 ... ## $ : num [1:75] -1.81 -1.652 -2.138 -0.816 -2.304 ... ## $ : num [1:80] -1.747 -2.63 -1.113 -2.78 -0.929 ... ## $ : num [1:85] -2.21 -1.85 -2.64 -0.85 -1.15 ... ## $ : num [1:90] -2.881 -0.366 -0.616 -2.621 -2.1 ... ## $ : num [1:95] -0.416 -2.188 -2.981 -1.865 -2.759 ... ## $ : num [1:100] -1.1 -1.33 -2.38 -3.78 -2.04 ... ## $ : num [1:105] -1.52 -3.03 -1.24 -2.55 -1.31 ... ## $ : num [1:110] -1.84 -1.99 -2.39 -3.7 -2.22 ... ## $ : num [1:115] -2.61 -1.77 -1.53 -1.49 -2.08 ... ## $ : num [1:120] -0.144 -2.653 -2.151 -2.956 -2.816 ... ## $ : num [1:125] -0.713 -1.665 -1.942 -0.271 -3.397 ... ## $ : num [1:130] -4.212 -2.855 -0.891 -1.408 -2.459 ... ## $ : num [1:135] -2.13 -3.3 -2.81 -1.45 -1.08 ... ## $ : num [1:140] -0.885 -1.899 -1.883 -2.05 -2.224 ... ## $ : num [1:145] -1.568 -2.834 -1.103 -0.573 -0.326 ... ## $ : num [1:150] -2.769 -3.933 -2.555 -2.899 -0.652 ... ## $ : num [1:5] -2.15 -1.455 -0.923 -2.791 -1.041 ## $ : num [1:10] -0.785 -3.017 -1.327 -2.766 -2.03 ... ## $ : num [1:15] -0.594 -4.592 -2.286 -2.583 -2.246 ... ## $ : num [1:20] -1.821 -0.527 -1.11 -2.759 -3.365 ... ## $ : num [1:25] 1.185 -3.176 -1.88 -0.506 -1.392 ... ## $ : num [1:30] -0.7988 -0.0641 -1.4638 -1.6671 -3.3243 ... ## $ : num [1:35] -3.35 -4.02 -1.15 -1.66 -3.27 ... ## $ : num [1:40] -1.625 -3.416 -2.233 -0.807 -1.334 ... ## $ : num [1:45] -2.36 -3.14 -2.76 -2 -3.15 ... ## $ : num [1:50] -2.06 -1.3 -1.75 -2.38 -1.2 ... ## $ : num [1:55] -0.916 -2.838 -3.182 -2.224 1.434 ... ## $ : num [1:60] -2.63 -1.46 -3.83 -2.16 -1.79 ... ## $ : num [1:65] -1.15 -2.72 -2.21 -1.86 -2.05 ... ## $ : num [1:70] -1.19 -2.71 -2.11 -1.3 -1.53 ... ## $ : num [1:75] -1.489 -1.233 -0.523 -3.204 -1.966 ... ## $ : num [1:80] -2.38 -2.283 -0.325 -1.147 -2.292 ... ## $ : num [1:85] -1.71 -1.63 -1.29 -1.17 -2.08 ... ## $ : num [1:90] -3.48 -1.1 -1.89 -1.68 -3.77 ... ## $ : num [1:95] -1.849 0.0107 -2.6893 -2.5238 -0.0639 ... ## $ : num [1:100] -1.694 -0.288 -1.509 -1.837 -2.627 ... ## $ : num [1:105] -2.43 -1.82 -1.37 -2.04 -1.75 ... ## $ : num [1:110] -1.6 -1.97 -2.35 -2.4 -3.82 ... ## $ : num [1:115] -2.38 -1.11 -2.35 -3.95 -2.17 ... ## $ : num [1:120] -2.354 -3.289 -1.679 -1.972 -0.384 ... ## $ : num [1:125] -1.79 -3.03 -2.01 -1.63 -1.98 ... ## $ : num [1:130] -1.04 -1.63 -1.75 -1.75 -1.76 ... ## $ : num [1:135] -1.02 -2.44 -1.24 -1.82 -2.9 ... ## $ : num [1:140] -1.947 -2.696 -1.286 -0.904 -0.126 ... ## $ : num [1:145] -3.4 -1.82 -1.34 -2.3 -1.56 ... ## $ : num [1:150] -2.07 -2.67 -2.49 -2.13 -2.04 ... ## $ : num [1:5] -0.0561 -0.7266 -0.989 -1.0046 -3.0633 ## $ : num [1:10] -2.217 -1.59 -2.841 -0.508 -1.318 ... ## $ : num [1:15] -2.38 -1.84 -2.28 -1.31 -1.88 ... ## $ : num [1:20] -0.987 -3.491 -1.836 -3.222 -2.567 ... ## $ : num [1:25] -0.276 -0.783 0.238 -0.226 -0.978 ... ## $ : num [1:30] -1.59 -2.08 -2.84 -1.74 -1.51 ... ## $ : num [1:35] -2.21 -3.17 -2.23 -1.94 -1.81 ... ## $ : num [1:40] -0.4 -3.42 -0.924 -0.348 -1.034 ... ## $ : num [1:45] -1.71 1.07 1.27 -1.54 -1.88 ... ## $ : num [1:50] -2.66 -1.044 -2.125 -0.914 -2.567 ... ## $ : num [1:55] -3.11 -1.768 -2.062 -1.028 -0.546 ... ## $ : num [1:60] -0.883 -0.711 -4.1 -1.876 -2.073 ... ## $ : num [1:65] -3.14513 -2.7894 -1.86605 0.00441 -0.17555 ... ## $ : num [1:70] -1.466 -1.795 -0.643 -1.793 -2.022 ... ## $ : num [1:75] -0.513 -3.371 -2.01 -0.451 -1.495 ... ## $ : num [1:80] -1.08 -2.33 -1.28 -3.05 -1.09 ... ## $ : num [1:85] -0.50768 -2.30933 -0.00181 -1.80426 -0.44882 ... ## $ : num [1:90] -1.534 -0.365 -2.928 -1.573 -3.251 ... ## $ : num [1:95] -0.768 -1.619 -1.675 -1.523 -3.053 ... ## $ : num [1:100] -3.19 -1.14 -0.29 -1.06 -2.91 ... ## $ : num [1:105] -0.8324 -2.8997 -2.1596 -2.0736 -0.0653 ... ## $ : num [1:110] -2.41 -1.17 -1.18 -3.23 -1.41 ... ## $ : num [1:115] -1.41 -1.59 -1.53 -2.86 -0.78 ... ## $ : num [1:120] -0.995 -0.544 -1.598 -2.371 0.511 ... ## $ : num [1:125] -2.35 -1.29 -2.55 -2.75 -1.29 ... ## $ : num [1:130] -4.294 0.355 -1.556 0.801 -0.822 ... ## $ : num [1:135] -2.843 -1.701 -0.396 -0.291 -1.538 ... ## $ : num [1:140] -2.283 -0.665 -1.567 -3.486 -2.585 ... ## $ : num [1:145] -0.0606 -0.2198 -1.5754 -2.0383 -2.4226 ... ## $ : num [1:150] -1.752 -2.476 -1.458 -1.395 0.188 ... ## $ : num [1:5] 0.948 1.077 -0.292 0.306 -1.144 ## $ : num [1:10] -0.481 -2.02 -1.348 -1.668 -0.986 ... ## $ : num [1:15] -1.196 1.02 -0.548 -0.916 -0.943 ... ## $ : num [1:20] -1.504 -1.351 -0.906 -2.073 -0.998 ... ## $ : num [1:25] -1.94 -1.8 -2.18 -2.4 -3 ... ## $ : num [1:30] -0.651 -0.9 -0.406 -2.767 -0.995 ... ## $ : num [1:35] -0.212 -2.165 -0.775 -0.564 -0.582 ... ## $ : num [1:40] -0.22 -0.982 -0.99 -0.411 -0.471 ... ## $ : num [1:45] 0.247 -2.077 -0.266 -0.624 0.473 ... ## [list output truncated] ``` --- # Simpler ### Maybe a little too clever * A data frame is a list so... ```r fsim <- pmap( full_conditions, ~rnorm(n = ..1, mean = ..2, sd = ..3) ) str(fsim) ``` ``` ## List of 10710 ## $ : num [1:5] -2.28 -1.9 -2.7 -3.14 -1.78 ## $ : num [1:10] -1.21 -3.23 -0.97 -1.28 -2.06 ... ## $ : num [1:15] -2.07 -1.24 -1.65 -0.27 -1.99 ... ## $ : num [1:20] -1.772 -2.843 -0.871 -1.46 -0.763 ... ## $ : num [1:25] 0.685 -0.119 -2.856 -0.688 -1.409 ... ## $ : num [1:30] -1.743 -2.922 -1.887 -2.03 -0.667 ... ## $ : num [1:35] -0.723 -1.828 -2.902 -0.284 -0.931 ... ## $ : num [1:40] -2.258 -3.35 -0.359 -0.794 -1.552 ... ## $ : num [1:45] -1.48 -1.92 -2.06 -1.99 -2.11 ... ## $ : num [1:50] -1.77 -1.84 -1.22 -1.44 -1.53 ... ## $ : num [1:55] -2.09 -2.6 -2.34 -3.12 -2.65 ... ## $ : num [1:60] -0.874 -0.363 -1.584 -1.647 -0.697 ... ## $ : num [1:65] -2.614 0.0316 -2.2096 -1.3851 -2.8877 ... ## $ : num [1:70] -1.833 -3.959 -1.424 -0.366 -3.91 ... ## $ : num [1:75] -2.525 -3.851 -2.547 -0.777 -1.011 ... ## $ : num [1:80] -1.974 -0.704 -3.198 -2.399 -2.608 ... ## $ : num [1:85] -3.173 -2.2 -2.965 -0.122 -2.95 ... ## $ : num [1:90] -1.13 -3.17 -1.88 -1.2 -3.8 ... ## $ : num [1:95] -1.862 -2.626 -0.544 -2.125 -2.76 ... ## $ : num [1:100] -1.386 -1.704 1.781 -0.196 -0.76 ... ## $ : num [1:105] -2.105 -2.706 -0.952 -1.631 -0.158 ... ## $ : num [1:110] -1.68 -2.81 -3.05 -1.12 -2.03 ... ## $ : num [1:115] -1.1 -1.73 -3.13 -2.96 -1.22 ... ## $ : num [1:120] -1.4 -2.73 -2.03 -2.72 -1.74 ... ## $ : num [1:125] -1.89 -1.52 -3.05 -1.38 -1.34 ... ## $ : num [1:130] -2.283 -1.87 -0.643 -3.037 -2.963 ... ## $ : num [1:135] -3.12 -1.28 -2.57 -2.12 -4.4 ... ## $ : num [1:140] -1.536 0.517 -1.5 -1.554 -3.014 ... ## $ : num [1:145] -2.711 -4.006 -2.072 -0.645 -3.781 ... ## $ : num [1:150] -0.577 -1.987 -2.5 -1.292 -1.443 ... ## $ : num [1:5] -2.178 -2.156 -0.833 -0.68 -3.071 ## $ : num [1:10] -2.572 -2.861 -2.25 -0.192 -0.631 ... ## $ : num [1:15] -2.4465 -1.2752 0.0537 -3.473 -3.5255 ... ## $ : num [1:20] -2.311 -1.859 -1.169 -0.695 -0.355 ... ## $ : num [1:25] -2.249 -1.955 -3.404 -0.827 -1.223 ... ## $ : num [1:30] -1.084 -0.819 -2.507 -0.789 -0.759 ... ## $ : num [1:35] -2.541 -0.313 -3.554 -2.763 -3.561 ... ## $ : num [1:40] -1.331 -1.798 -1.66 -1.539 -0.686 ... ## $ : num [1:45] -2.027 -2.526 -0.529 -3.618 -1.236 ... ## $ : num [1:50] -2.07 -2.05 -1.5 -3.21 -2.03 ... ## $ : num [1:55] -1.22 -1.924 -1.446 -1.52 -0.958 ... ## $ : num [1:60] -0.385 -2.353 -3.338 -2.524 -0.507 ... ## $ : num [1:65] -1.84 -2.296 -1.053 -0.787 -1.104 ... ## $ : num [1:70] -1.003 -2.191 -1.335 0.158 -2.166 ... ## $ : num [1:75] -3.445 -0.219 -2.692 -0.65 -2.058 ... ## $ : num [1:80] -1.504 -0.545 -2.231 -0.995 -1.946 ... ## $ : num [1:85] -3.095 -2.434 -1.092 -1.478 -0.942 ... ## $ : num [1:90] -0.406 0.405 -2.241 -3.095 -0.688 ... ## $ : num [1:95] -2.082 -2.501 -2.125 -3.098 -0.545 ... ## $ : num [1:100] -1.99 -2.18 -2.76 -1.23 -1.3 ... ## $ : num [1:105] -0.623 -2.443 -2.883 -0.733 -1.265 ... ## $ : num [1:110] -1.79 -2.23 -1.67 -1.55 -1.65 ... ## $ : num [1:115] -0.494 -2.707 -1.158 0.198 -1.645 ... ## $ : num [1:120] -1.702 -1.82 -0.179 -2.122 -1.1 ... ## $ : num [1:125] -2.89 -1.72 -2.62 -1.56 -1.34 ... ## $ : num [1:130] -0.707 -2.066 -2.257 -2.559 -1.533 ... ## $ : num [1:135] -1.18 -1.2 -2.54 -1.17 -1.15 ... ## $ : num [1:140] -1.019 -2.102 -0.813 -1.88 -1.331 ... ## $ : num [1:145] -2.88 -2.42 -3.67 -2.83 -1.69 ... ## $ : num [1:150] -0.95403 -2.71064 -0.00388 -2.28287 -1.99366 ... ## $ : num [1:5] -2.299 -0.449 -1.123 -0.322 -0.712 ## $ : num [1:10] -2.422 -2.682 -0.663 -1.59 -0.312 ... ## $ : num [1:15] -2.198 0.276 -0.194 0.243 -3.398 ... ## $ : num [1:20] -2.75 -1.3 -2.59 -1.54 -2.53 ... ## $ : num [1:25] -2.46 -1.37 -2.98 -2.17 -2.64 ... ## $ : num [1:30] -1.06 0.795 -1.722 -2.103 -1.231 ... ## $ : num [1:35] -2.395 -2.373 -0.539 -2.531 -1.835 ... ## $ : num [1:40] -0.507 -1.933 -2.315 -1.173 -1.324 ... ## $ : num [1:45] -3.574 -1.158 -1.389 -3.243 -0.358 ... ## $ : num [1:50] 0.0716 -1.3999 -0.297 -2.9978 -1.5942 ... ## $ : num [1:55] -1.662 -1.894 -0.745 -4.105 -0.771 ... ## $ : num [1:60] -2.72 -3.065 -0.775 -1.59 -1.514 ... ## $ : num [1:65] -2.33 -1.55 -2.45 -2.9 -3.22 ... ## $ : num [1:70] -1.6414 -2.4184 -3.1205 -1.1381 -0.0615 ... ## $ : num [1:75] -1.29 -2.19 -1.86 -2.06 -2.54 ... ## $ : num [1:80] -0.973 -1.99 -1.385 -0.765 -2.101 ... ## $ : num [1:85] -1.487 -1.185 -1.066 -0.859 -1.014 ... ## $ : num [1:90] -3.153 -0.197 -1.399 0.484 -1.939 ... ## $ : num [1:95] -0.694 -0.905 -0.984 0.813 -1.924 ... ## $ : num [1:100] -1.617 -1.874 -2.269 -0.855 -2.222 ... ## $ : num [1:105] -1.039 -1.863 -3.459 -1.276 0.671 ... ## $ : num [1:110] -2.133 -3.227 0.253 -1.422 -0.769 ... ## $ : num [1:115] -2.301 -2.512 -0.254 -1.807 -1.687 ... ## $ : num [1:120] -3.052 -2.437 0.492 -1.163 -1.715 ... ## $ : num [1:125] -2.382 -2.207 -0.729 -1.653 -0.544 ... ## $ : num [1:130] -1.952 1.473 -2.208 -0.636 -0.921 ... ## $ : num [1:135] -0.168 -2.362 -2.218 -2.683 -0.998 ... ## $ : num [1:140] 0.579 -3.581 -2.371 -1.968 -1.829 ... ## $ : num [1:145] -0.9203 -1.2718 -2.3872 -2.2341 0.0542 ... ## $ : num [1:150] -0.802 -0.22 -1.189 -2.149 -2.411 ... ## $ : num [1:5] -0.441 -2.207 -1.598 -1.914 -1.844 ## $ : num [1:10] -0.583 -0.916 -0.504 -0.158 1.169 ... ## $ : num [1:15] -3.725 -2.738 -1.077 -0.886 -0.747 ... ## $ : num [1:20] -1.288 -1.995 -2.593 -1.747 0.514 ... ## $ : num [1:25] -0.691 -2.092 -1.742 -2.113 -0.812 ... ## $ : num [1:30] -0.891 0.793 -0.14 -1.519 -0.837 ... ## $ : num [1:35] -1.966 -1.311 -0.326 -1.336 -0.674 ... ## $ : num [1:40] -1.98 -1.78 -1.82 -1.76 -3.3 ... ## $ : num [1:45] 0.236 -1.342 -1.601 -1.899 -1.537 ... ## [list output truncated] ``` --- # List column version ```r full_conditions %>% as_tibble() %>% mutate(sim = pmap(list(n, mu, sd), ~rnorm(..1, ..2, ..3))) ``` ``` ## # A tibble: 10,710 x 4 ## n mu sd sim ## <dbl> <dbl> <dbl> <list> ## 1 5 -2 1 <dbl [5]> ## 2 10 -2 1 <dbl [10]> ## 3 15 -2 1 <dbl [15]> ## 4 20 -2 1 <dbl [20]> ## 5 25 -2 1 <dbl [25]> ## 6 30 -2 1 <dbl [30]> ## 7 35 -2 1 <dbl [35]> ## 8 40 -2 1 <dbl [40]> ## 9 45 -2 1 <dbl [45]> ## 10 50 -2 1 <dbl [50]> ## # … with 10,700 more rows ``` --- # Unnest ```r full_conditions %>% as_tibble() %>% mutate(sim = pmap(list(n, mu, sd), ~rnorm(..1, ..2, ..3))) %>% unnest(sim) ``` ``` ## # A tibble: 830,025 x 4 ## n mu sd sim ## <dbl> <dbl> <dbl> <dbl> ## 1 5 -2 1 -0.9769357 ## 2 5 -2 1 -1.026245 ## 3 5 -2 1 -1.983785 ## 4 5 -2 1 -1.262065 ## 5 5 -2 1 -0.3616705 ## 6 10 -2 1 -1.136676 ## 7 10 -2 1 -1.665104 ## 8 10 -2 1 -3.062858 ## 9 10 -2 1 -4.412271 ## 10 10 -2 1 -0.9440350 ## # … with 830,015 more rows ``` --- # Replicate with `nest_by()` ### You try first
02
:
00
-- ```r full_conditions %>% rowwise() %>% mutate(sim = list(rnorm(n, mu, sd))) %>% unnest(sim) ``` ``` ## # A tibble: 830,025 x 4 ## n mu sd sim ## <dbl> <dbl> <dbl> <dbl> ## 1 5 -2 1 -4.246447 ## 2 5 -2 1 -3.394033 ## 3 5 -2 1 -2.688046 ## 4 5 -2 1 -4.004150 ## 5 5 -2 1 -2.636363 ## 6 10 -2 1 -0.5402081 ## 7 10 -2 1 -2.075118 ## 8 10 -2 1 -1.927610 ## 9 10 -2 1 -2.871188 ## 10 10 -2 1 -0.9080542 ## # … with 830,015 more rows ``` --- class: inverse-blue middle # Plot Add a caption stating the total number of Pulitzer prize winners across years --- # Add column for total ```r pulitzer<- pulitzer%>% group_by(newspaper) %>% mutate(tot = sum(n)) pulitzer ``` ``` ## # A tibble: 100 x 4 ## # Groups: newspaper [50] ## newspaper year_range n tot ## <chr> <chr> <int> <int> ## 1 USA Today 1990-2003 1 2 ## 2 USA Today 2004-2014 1 2 ## 3 Wall Street Journal 1990-2003 30 50 ## 4 Wall Street Journal 2004-2014 20 50 ## 5 New York Times 1990-2003 55 117 ## 6 New York Times 2004-2014 62 117 ## 7 Los Angeles Times 1990-2003 44 85 ## 8 Los Angeles Times 2004-2014 41 85 ## 9 Washington Post 1990-2003 52 100 ## 10 Washington Post 2004-2014 48 100 ## # … with 90 more rows ``` --- # Easiest way (imo) Create a column to represent exactly the label you want. ```r #install.packages("english") library(english) pulitzer<- pulitzer%>% mutate( label = glue( "{str_to_title(as.english(tot))} Total Pulitzer Awards" ) ) ``` --- ```r select(pulitzer, newspaper, label) ``` ``` ## # A tibble: 100 x 2 ## # Groups: newspaper [50] ## newspaper label ## <chr> <glue> ## 1 USA Today Two Total Pulitzer Awards ## 2 USA Today Two Total Pulitzer Awards ## 3 Wall Street Journal Fifty Total Pulitzer Awards ## 4 Wall Street Journal Fifty Total Pulitzer Awards ## 5 New York Times One Hundred Seventeen Total Pulitzer Awards ## 6 New York Times One Hundred Seventeen Total Pulitzer Awards ## 7 Los Angeles Times Eighty-Five Total Pulitzer Awards ## 8 Los Angeles Times Eighty-Five Total Pulitzer Awards ## 9 Washington Post One Hundred Total Pulitzer Awards ## 10 Washington Post One Hundred Total Pulitzer Awards ## # … with 90 more rows ``` --- # Produce one plot ```r tmp <- pulitzer%>% filter(newspaper == "Wall Street Journal") ggplot(tmp, aes(n, year_range)) + geom_col(aes(fill = n)) + scale_fill_distiller(type = "seq", limits = c(0, max(pulitzer$n)), palette = "BuPu", direction = 1) + scale_x_continuous(limits = c(0, max(pulitzer$n)), expand = c(0, 0)) + guides(fill = "none") + labs( title = glue("Pulitzer Prize winners: Wall Street Journal"), x = "Total number of winners", y = "", caption = unique(tmp$label) ) ``` --- class: middle 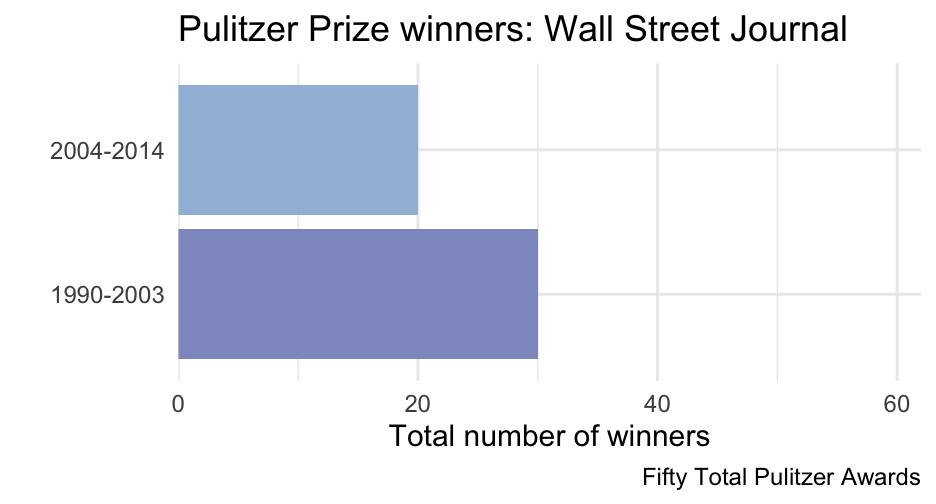<!-- --> --- # Produce all plots ### Nest first ```r pulitzer%>% group_by(newspaper, label) %>% nest() ``` ``` ## # A tibble: 50 x 3 ## # Groups: newspaper, label [50] ## newspaper label data ## <chr> <glue> <list> ## 1 USA Today Two Total Pulitzer Awards <tibble[,3] [2 × 3]> ## 2 Wall Street Journal Fifty Total Pulitzer Awards <tibble[,3] [2 × 3]> ## 3 New York Times One Hundred Seventeen Total Pulitzer Awards <tibble[,3] [2 × 3]> ## 4 Los Angeles Times Eighty-Five Total Pulitzer Awards <tibble[,3] [2 × 3]> ## 5 Washington Post One Hundred Total Pulitzer Awards <tibble[,3] [2 × 3]> ## 6 New York Daily News Six Total Pulitzer Awards <tibble[,3] [2 × 3]> ## 7 New York Post Zero Total Pulitzer Awards <tibble[,3] [2 × 3]> ## 8 Chicago Tribune Thirty-Eight Total Pulitzer Awards <tibble[,3] [2 × 3]> ## 9 San Jose Mercury News Six Total Pulitzer Awards <tibble[,3] [2 × 3]> ## 10 Newsday Eighteen Total Pulitzer Awards <tibble[,3] [2 × 3]> ## # … with 40 more rows ``` --- # Produce plots ```r final_plots <- pulitzer%>% group_by(newspaper, label) %>% nest() %>% mutate(plots = pmap(list(newspaper, label, data), ~{ * ggplot(..3, aes(n, year_range)) + geom_col(aes(fill = n)) + scale_fill_distiller(type = "seq", limits = c(0, max(pulitzer$n)), palette = "BuPu", direction = 1) + scale_x_continuous(limits = c(0, max(pulitzer$n)), expand = c(0, 0)) + guides(fill = "none") + * labs(title = glue("Pulitzer Prize winners: {..1}"), x = "Total number of winners", y = "", * caption = ..2) }) ) ``` --- # Look at a couple plots .pull-left[ ```r final_plots$plots[[1]] ``` 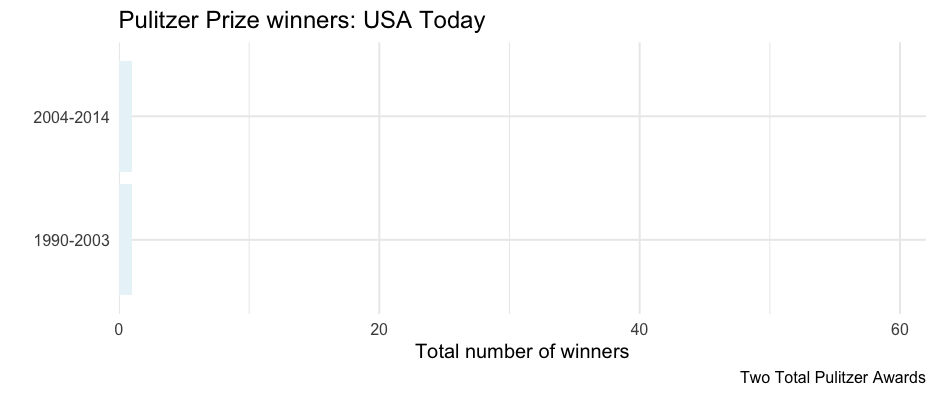<!-- --> ```r final_plots$plots[[3]] ``` 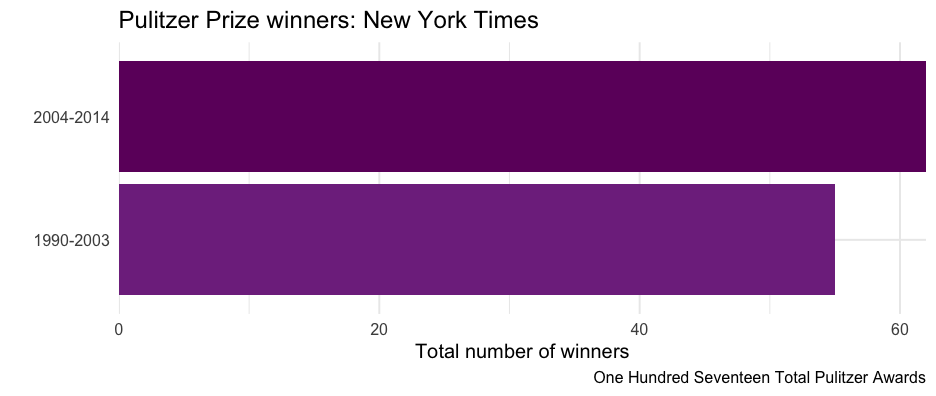<!-- --> ] .pull-right[ ```r final_plots$plots[[2]] ``` 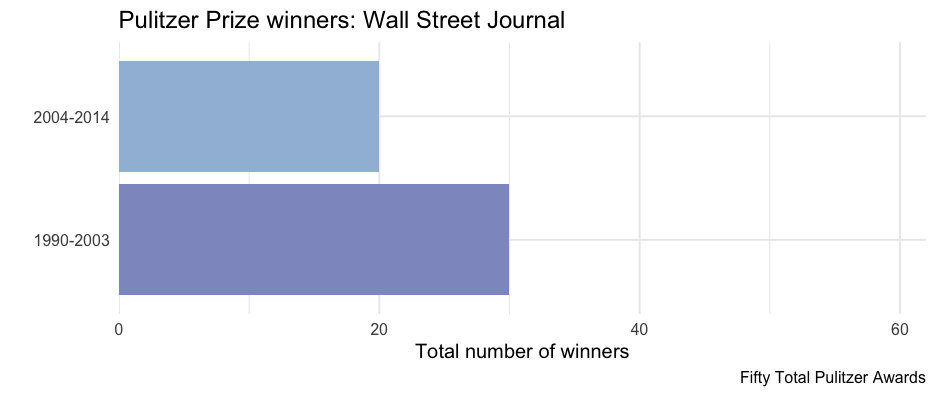<!-- --> ```r final_plots$plots[[4]] ``` 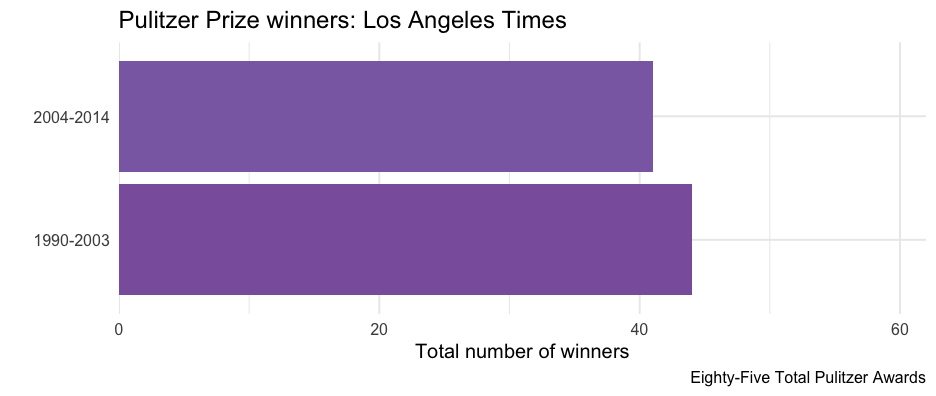<!-- --> ] --- # Replicate with `nest_by()` You try first
03
:
00
--- ```r final_plots2 <- pulitzer%>% ungroup() %>% nest_by(newspaper, label) %>% mutate( plots = list( ggplot(data, aes(n, year_range)) + geom_col(aes(fill = n)) + scale_fill_distiller(type = "seq", limits = c(0, max(pulitzer$n)), palette = "BuPu", direction = 1) + scale_x_continuous(limits = c(0, max(pulitzer$n)), expand = c(0, 0)) + guides(fill = "none") + labs(title = glue("Pulitzer Prize winners: {newspaper}"), x = "Total number of winners", y = "", caption = label) ) ) ``` --- ```r final_plots2 ``` ``` ## # A tibble: 50 x 4 ## # Rowwise: newspaper, label ## newspaper label data plots ## <chr> <glue> <list<tibble[,3]>> <list> ## 1 Arizona Republic Seven Total Pulitzer Awards [2 × 3] <gg> ## 2 Atlanta Journal Constitution Six Total Pulitzer Awards [2 × 3] <gg> ## 3 Baltimore Sun Thirteen Total Pulitzer Awards [2 × 3] <gg> ## 4 Boston Globe Forty-One Total Pulitzer Awards [2 × 3] <gg> ## 5 Boston Herald Zero Total Pulitzer Awards [2 × 3] <gg> ## 6 Charlotte Observer Four Total Pulitzer Awards [2 × 3] <gg> ## 7 Chicago Sun-Times Two Total Pulitzer Awards [2 × 3] <gg> ## 8 Chicago Tribune Thirty-Eight Total Pulitzer Awards [2 × 3] <gg> ## 9 Cleveland Plain Dealer Eleven Total Pulitzer Awards [2 × 3] <gg> ## 10 Columbus Dispatch One Total Pulitzer Awards [2 × 3] <gg> ## # … with 40 more rows ``` --- # Save all plots We'll have to iterate across at least two things: (a) file path/names, and (b) the plots themselves -- We can do this with the `map()` family, but instead we'll use a different function, which we'll talk about more next week. -- As an aside, what are the **steps** we would need to take to do this? -- Could we use a `nest_by()` solution? --- # Try with `nest_by()` You try first: * Create a vector of file paths * "loop" through the file paths and the plots to save them
04
:
00
--- # Example ### Create a directory ```r fs::dir_create(here::here("plots", "pulitzers")) ``` -- ### Create file paths ```r files <- str_replace_all(tolower(final_plots$newspaper), " ", "-") paths <- here::here("plots", "pulitzers", glue("{files}.png")) paths ``` ``` ## [1] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/usa-today.png" ## [2] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/wall-street-journal.png" ## [3] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/new-york-times.png" ## [4] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/los-angeles-times.png" ## [5] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/washington-post.png" ## [6] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/new-york-daily-news.png" ## [7] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/new-york-post.png" ## [8] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/chicago-tribune.png" ## [9] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/san-jose-mercury-news.png" ## [10] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/newsday.png" ## [11] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/houston-chronicle.png" ## [12] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/dallas-morning-news.png" ## [13] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/san-francisco-chronicle.png" ## [14] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/arizona-republic.png" ## [15] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/chicago-sun-times.png" ## [16] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/boston-globe.png" ## [17] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/atlanta-journal-constitution.png" ## [18] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/newark-star-ledger.png" ## [19] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/detroit-free-press.png" ## [20] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/minneapolis-star-tribune.png" ## [21] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/philadelphia-inquirer.png" ## [22] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/cleveland-plain-dealer.png" ## [23] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/san-diego-union-tribune.png" ## [24] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/tampa-bay-times.png" ## [25] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/denver-post.png" ## [26] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/rocky-mountain-news.png" ## [27] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/oregonian.png" ## [28] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/miami-herald.png" ## [29] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/orange-county-register.png" ## [30] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/sacramento-bee.png" ## [31] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/st.-louis-post-dispatch.png" ## [32] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/baltimore-sun.png" ## [33] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/kansas-city-star.png" ## [34] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/detroit-news.png" ## [35] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/orlando-sentinel.png" ## [36] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/south-florida-sun-sentinel.png" ## [37] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/new-orleans-times-picayune.png" ## [38] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/columbus-dispatch.png" ## [39] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/indianapolis-star.png" ## [40] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/san-antonio-express-news.png" ## [41] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/pittsburgh-post-gazette.png" ## [42] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/milwaukee-journal-sentinel.png" ## [43] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/tampa-tribune.png" ## [44] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/fort-woth-star-telegram.png" ## [45] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/boston-herald.png" ## [46] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/seattle-times.png" ## [47] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/charlotte-observer.png" ## [48] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/daily-oklahoman.png" ## [49] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/louisville-courier-journal.png" ## [50] "/Users/daniel/Teaching/data_sci_specialization/2020-21/c3-fp-2021/plots/pulitzers/investor's-buisiness-daily.png" ``` --- # Add paths to data frame ```r final_plots %>% * ungroup() %>% mutate(path = paths) %>% select(plots, path) ``` ``` ## # A tibble: 50 x 2 ## plots ## <list> ## 1 <gg> ## 2 <gg> ## 3 <gg> ## 4 <gg> ## 5 <gg> ## 6 <gg> ## 7 <gg> ## 8 <gg> ## 9 <gg> ## 10 <gg> ## # … with 40 more rows, and 1 more variable: path <chr> ``` --- # Save ```r final_plots %>% * ungroup() %>% mutate(path = paths) %>% * rowwise() %>% summarize( ggsave( path, plots, width = 9.5, height = 6.5, dpi = 500 ) ) ``` ``` ## # A tibble: 50 x 0 ``` --- # Wrap-up * Parallel iterations greatly increase the things you can do - iterating through at least two things simultaneously is pretty common -- * The `nest_by()` approach can regularly get you the same result as `group_by() %>% nest() %>% mutate() %>% map()` + Caveat - must be in a data frame, which means working with list columns -- * My view - it's still worth learning both. Looping with **{purrr}** is super flexible and often safer than base versions (type safe). Doesn't have to be used within a data frame. --- class: inverse-green middle # Next time ### Looping variants